What is Paylocity?
Paylocity is an human capital management (HCM) that offers a comprehensive suite of HR solutions designed to streamline payroll processing, enhance employee engagement, and improve overall workforce management.
In this guide, we’ll walk you through how to set up and integrate the Paylocity API into your system, from authentication to handling common post-integration issues.
Note : If you’re looking for an easier way to launch integrations with Paylocity, Bindbee can help. Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, whether you're a developer integrating HR functions or syncing payroll data, this guide will provide the technical steps and practical insights you need.
Why Integrate Paylocity API?
For developers, the ability to sync data between Paylocity and other platforms is essential for delivering a cohesive experience to your customers.
By integrating with Paylocity API, you enable automated data exchange to access data across employee records, payroll, and benefits without manual intervention.
With these benefits in mind, let’s dive into the technical process of integrating Paylocity API.
Setting Up Paylocity API Authentication
The first step in any API integration is authentication.
Paylocity uses OAuth 2.0 for secure access, which means you’ll need to generate access tokens to make API requests.
Step-by-Step Authentication Guide
- Get Client Credentials: Obtain your client ID and secret from Paylocity. This will be used to authenticate your API requests.
- Request Access Token:Use the following example to request an access token from Paylocity’s OAuth server.
import requests
url = "<https://api.paylocity.com/oauth/token>"
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
}
data = {
'grant_type': 'client_credentials',
'client_id': 'your-client-id',
'client_secret': 'your-client-secret'
}
response = requests.post(url, headers=headers, data=data)
access_token = response.json()['access_token']
This token will be needed for all subsequent API requests.
3. Handle Token Expiration: Tokens expire after a set period. Implement logic to refresh the token automatically when expired to avoid interruptions in API communication.
Example of Token Refresh Logic
Here’s how you can automate this process:
def refresh_token():
data = {
'grant_type': 'refresh_token',
'refresh_token': 'your-refresh-token',
'client_id': 'your-client-id',
'client_secret': 'your-client-secret'
}
response = requests.post(url, headers=headers, data=data)
return response.json()['access_token']
Exploring Key Paylocity API Endpoints
Once authenticated, you’ll need to understand the core endpoints that you’ll interact with. These endpoints are important for syncing employee data, payroll, and timesheets.
1. Employee Data
The most commonly used endpoints for managing employee data include creating, updating, and fetching employee information.
Here’s an example of how to fetch employee details:
url = "<https://api.paylocity.com/v1/employee/{employeeId}>"
headers = {
'Authorization': f'Bearer {access_token}'
}
response = requests.get(url, headers=headers)
employee_data = response.json()
2. Payroll Processing
To automate payroll, Paylocity’s API allows you to access payroll data and submit payroll changes directly. Example endpoints include:
GET /v1/payroll/run/{runId}
: Retrieve details of a specific payroll run.POST /v1/payroll/adjustments
: Make adjustments to a payroll run.
3. Benefits and Timekeeping
You can also manage employee benefits and track working hours via the Paylocity API.
Fetching and Managing Employee Data
Data management is a crucial part of any HR-Tech integration.
Given the volume of employee records that might need syncing, it’s important to handle large datasets effectively.
Handling Pagination
When dealing with a large employee base, data responses from the API might be paginated. Make sure to check the response for pagination details and fetch subsequent pages where necessary.
def fetch_paginated_data(url, headers):
data = []
while url:
response = requests.get(url, headers=headers)
data.extend(response.json()['results'])
url = response.json().get('next') # Get next page URL
return data
Best Practices for Managing Large Datasets
- Optimize API Calls: When dealing with large datasets, use pagination and filters to minimize the data load on your system.
- Monitor API Rate Limits: Keep track of Paylocity’s API rate limits and plan accordingly to avoid interruptions in data syncing.
Use Cases for Paylocity API Integration
1. Automating Payroll Processing
A common use case for the Paylocity API is automating payroll. Using the API, you can automatically send payroll data to Paylocity, calculate taxes, and manage deductions, all without human input.
2. Employee Data Syncing
For Tech vendors, integrating employee data is crucial. By using Paylocity’s API, you can keep employee records up to date, reflecting changes in position, salary, or benefits in real-time.
3. Benefits Management Integration
Synchronizing benefits data across systems helps eliminate manual errors and ensures employees receive the correct benefits. Paylocity’s API allows you to update and manage benefits programmatically.
Best Practices for Paylocity API Integration
To ensure a successful and efficient integration, here are some best practices:
- Data Security: Always use HTTPS when making API requests. Protect sensitive HR data by encrypting requests and responses.
- Error Handling: Build in error handling for failed API requests. Log errors and retry failed requests where appropriate.
- Testing in Sandbox: Before moving to production, thoroughly test your integration in Paylocity’s sandbox environment to catch potential issues.
Error Code Reference Table
A quick guide to common error codes and solutions:
Post-Integration Troubleshooting and Support
Even after a successful integration, issues can arise.
Here are some common problems and solutions:
Common Errors
- 401 Unauthorized: Ensure that your access token is valid and has not expired.
- Rate Limits: Paylocity enforces API rate limits. Implement retry logic to handle rate limit errors without losing data.
Monitoring and Debugging
Set up proper logging to monitor API requests and responses.
This will allow you to identify and troubleshoot errors quickly. Use tools like Postman or Curl to manually test endpoints when debugging.
Getting Started with Paylocity API using Bindbee
Integrating with Paylocity shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink, draining valuable engineering resources.
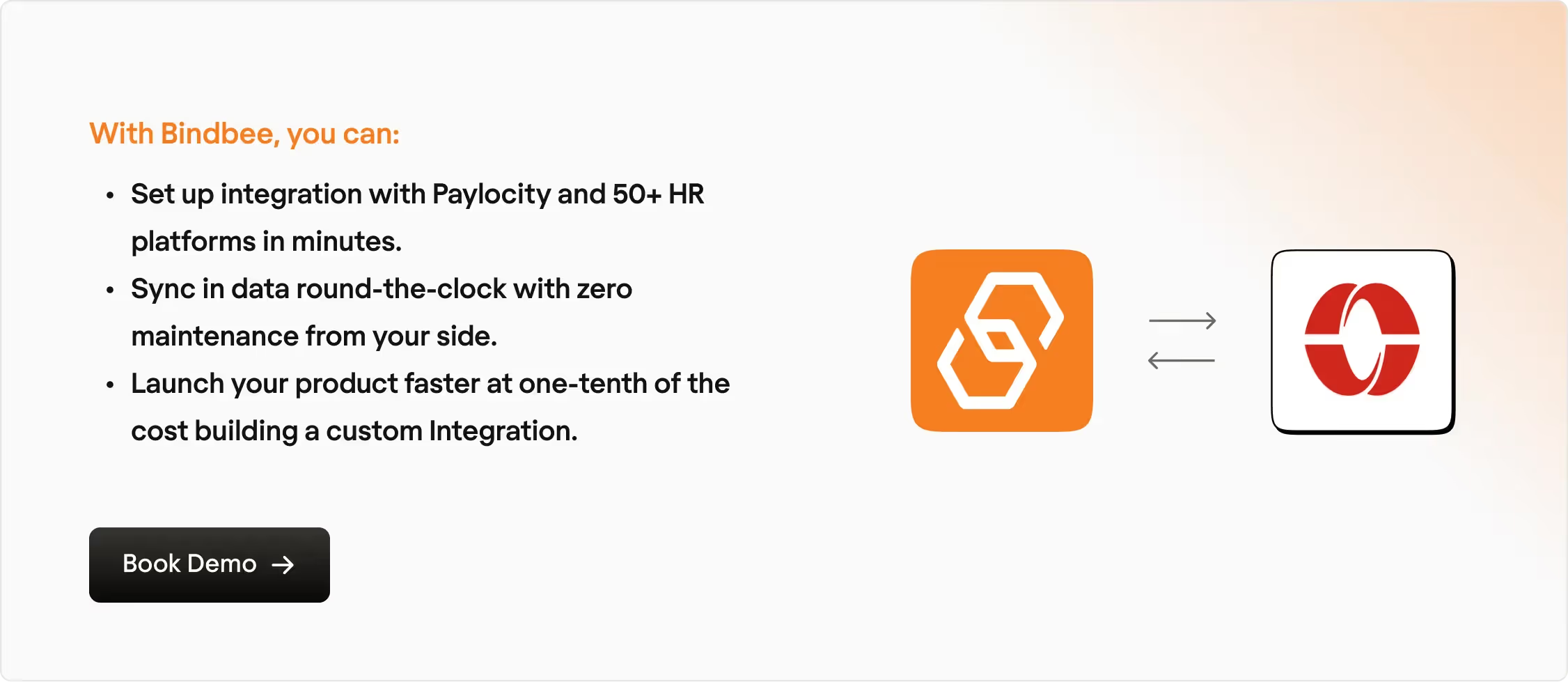
Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.
FAQ
- What is the API rate limit for Paylocity?
- Paylocity enforces rate limits to protect the system. Implement retry logic to handle these scenarios without losing data.
- How do I handle large employee datasets efficiently?
- Use pagination when fetching large datasets to optimize data syncing.
- What should I do if I encounter persistent 403 errors?
- Check your API permissions and ensure the correct scopes are assigned to your access tokens.