What is Bamboo HR?
BambooHR is an Human resources information system (HRIS) designed to help organizations manage employee information, payroll, benefits, and more.
In this guide, we’ll explore how to integrate BambooHR’s API into your applications, look at common use cases, and offer best practices for troubleshooting API issues. Whether you’re a developer, HR-tech professional, or part of a tech team evaluating BambooHR, this blog aims to help you leverage the API effectively.
Note - This is an educational article on Bamboo HR API. If you’re looking for an easier way to launch integrations with Bamboo HR, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
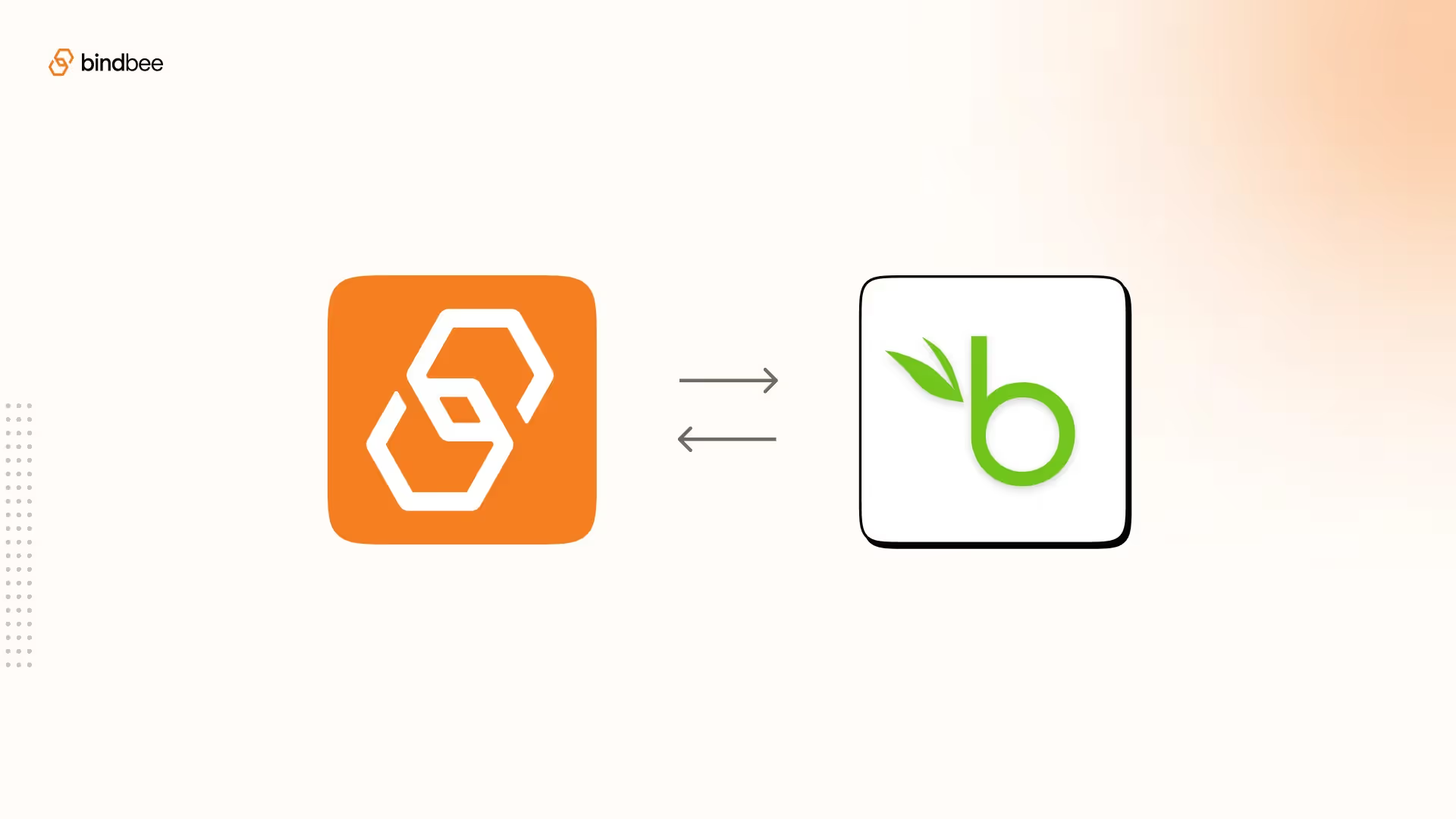
That said, Here’s what we’ll cover in this article:
- A step-by-step integration guide using Python, Postman, and Bindbee.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for smooth API operations.
Understanding BambooHR API
What is BambooHR API?
The BambooHR API is a RESTful service that allows you to access and manipulate BambooHR data programmatically.
This includes managing employee data, time-off requests, departments, and generating custom reports. With the API, you can integrate BambooHR data with other platforms like payroll systems, internal dashboards or to your own applications.
API Authentication
BambooHR API uses two authentication methods: API Keys and OAuth.
- API Keys: This is the most common method. You’ll need to generate an API key from your BambooHR account. This key acts as a password for your requests and should be included in the headers of your API calls.
- Example of authenticating using API key with
curl
:
curl -i -u "{API Key}:x" "<https://api.bamboohr.com/api/gateway.php/{subdomain}/v1/employees/directory>"
- OAuth: OAuth is useful for limiting data access based on predefined scopes. While OAuth is in limited rollout for BambooHR, it offers more granular control over what data can be accessed.
Handling API Requests
API requests follow the REST structure, meaning you’ll use HTTPS methods (GET, POST, PUT, DELETE) to interact with BambooHR’s data. Responses can be returned in JSON or XML, depending on your preference.
- Base URL:
<https://api.bamboohr.com/api/gateway.php/{subdomain}/>
BambooHR API Integration Guide
We’ll walk through three methodologies: Python, Postman, and Bindbee.
Whether you’re comfortable with code, prefer a GUI like Postman, or outsource the entire process to a unified API like Bindbee, this section has you covered.
Method 1: Python
Setup: First, install the requests
library and get your BambooHR API key from the account settings.
pip install requests
Retrieve Employee Data
Here’s how you can use Python to pull data for a specific employee.
import requests
url = "<https://api.bamboohr.com/api/gateway.php/{subdomain}/v1/employees/4/?fields=firstName,lastName>"
headers = {
"Accept": "application/json",
"Authorization": "Basic {encoded_api_key}"
}
response = requests.get(url, headers=headers)
print(response.json())
This code retrieves the employee’s first and last name.
You can expand the fields to include other information like job title, department, etc.
Bulk Employee Data (Custom Reports)
You can retrieve bulk data using the custom report endpoint.
url = "<https://api.bamboohr.com/api/gateway.php/{subdomain}/v1/reports/custom?format=JSON>"
payload = {"fields": ["firstName", "lastName", "jobTitle"]}
response = requests.post(url, json=payload, headers=headers)
print(response.json())
Error Handling
When interacting with the BambooHR API, you might encounter 401 Unauthorized or 403 Forbidden errors.
Make sure your API key is valid, and the user associated with it has the correct permissions.
Method 2: Postman
Setup Install Postman and create a new collection for BambooHR API requests. Set up Basic Auth using your API key.
- Create a collection and set Basic Auth under the Authorization tab.
- Enter your API key in the username field and any random string in the password field.
Retrieve Employee Data
Use the following setup in Postman to retrieve data for a specific employee.
- URL:
<https://api.bamboohr.com/api/gateway.php/{subdomain}/v1/employees/4>
- Params:
fields=firstName,lastName
- Authorization: Basic Auth
Send the request, and Postman will return the employee's first and last name.
Bulk Data Retrieval
To pull bulk employee data, use the custom reports endpoint.
- URL:
<https://api.bamboohr.com/api/gateway.php/{subdomain}/v1/reports/custom>
- Method: POST
- Params:
format=JSON
- Body: Raw JSON format with the required fields.
Method 3: Bindbee
Overview: Bindbee is a unified API platform that lets you integrate with Bamboo HR (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up BambooHR Integration with Bindbee
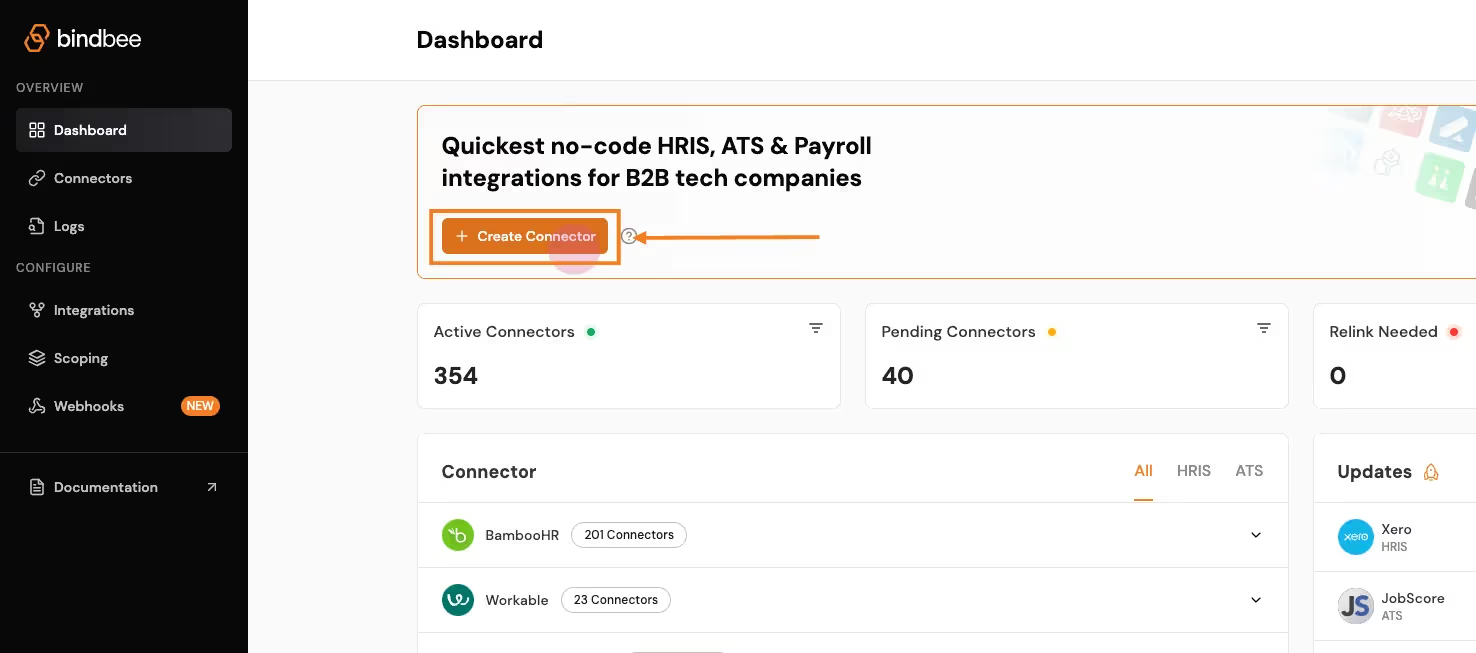
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., BambooHR_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with BambooHR.
- Open the link and enter the necessary credentials (e.g., BambooHR API key, subdomain). This step establishes the connection between BambooHR and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from BambooHR. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from BambooHR.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the BambooHR data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from BambooHR through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "Kunal",
"last_name": "Tyagi",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "kunal@bindbee.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from BambooHR.
The pagination feature helps manage large responses by returning results in pages.
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
BambooHR API: Common Use Cases
The BambooHR API is incredibly versatile. Here are some common use cases:
- HR Automation: Automate processes like employee onboarding and leave requests. For example, sync BambooHR data with payroll systems to ensure smooth employee payroll setups.
- Custom Reporting: Create custom reports to monitor employee performance or analyze turnover rates. The API allows you to pull detailed employee data and export it in different formats (CSV, PDF, etc.).
- Third-Party Integration: The API can be used to sync employee data with third-party tech vendors, ensuring accurate and up-to-data sync for faster Time-to-value.
Debugging and Best Practices
When working with APIs for BambooHR, you might encounter various errors during integration.
These issues typically arise from authentication, permissions, or rate limits.
Here's a breakdown of common errors, their causes, and solutions.
Best Practices for Efficient API Usage
To avoid common pitfalls and ensure your BambooHR integration performs efficiently, follow these guidelines:
- Efficient Data Syncing:
- Batch Requests: When retrieving large datasets, use bulk endpoints like custom reports to avoid making numerous API calls.
- Pagination: For large data sets, paginate responses to handle records in manageable batches. This ensures that your integration remains performant and within rate limits.
Example (using Bindbee):
url = "<https://api.bindbee.com/hris/v1/employees?page_size=50&cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1>"
response = requests.get(url, headers=headers)
- Handle Rate Limits Gracefully:
- Use HTTP headers to monitor rate limits. For example, BambooHR returns
Retry-After
headers when rate limits are exceeded, guiding you on when to retry. - Implement exponential backoff to delay retries progressively in case of transient errors (e.g., 429 Too Many Requests or 503 Service Unavailable).
- Use HTTP headers to monitor rate limits. For example, BambooHR returns
Example of exponential backoff:
import time
for i in range(5): # Retry up to 5 times
response = requests.get(url, headers=headers)
if response.status_code == 200:
break
elif response.status_code == 429:
retry_after = int(response.headers.get('Retry-After', 10))
time.sleep(retry_after * (2 ** i)) # Exponentially increase retry time
- Error Handling:
- Always check the status codes and handle errors appropriately. Use logging to capture errors for debugging.
- For critical errors like 401 Unauthorized or 403 Forbidden, alert the user immediately to correct the API key or permissions.
- Caching:
- For frequently accessed data, implement caching mechanisms to reduce repetitive API calls and improve performance. For example, cache the employee directory and refresh the cache periodically rather than fetching data on every request.
- Timeouts and Retries:
- Set a reasonable timeout for API requests to avoid hanging indefinitely. Implement retries with a timeout threshold to handle network issues or server delays gracefully.
Example:
response = requests.get(url, headers=headers, timeout=10) # Timeout after 10 seconds
- Use the Right HTTP Methods:
- Follow RESTful best practices by using the correct HTTP methods:
GET
for retrieving data.POST
for creating new resources.PUT
orPATCH
for updating existing resources.DELETE
for deleting resources.
- Follow RESTful best practices by using the correct HTTP methods:
- Monitoring and Alerts:
- Set up monitoring for your API integration to track response times, error rates, and downtime. This will help you identify potential issues before they impact users.
- Use tools like Postman or API monitoring platforms to regularly test and validate your API requests.
By applying these best practices and handling common errors effectively, your BambooHR integration will remain efficient and reliable.
6. BambooHR API Documentation and Resources
- BambooHR API Documentation
- Tools for testing and debugging:
- Bindbee’s API documentation.
Get Started with ADP API Using Bindbee
Integrating with Bamboo HR shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.
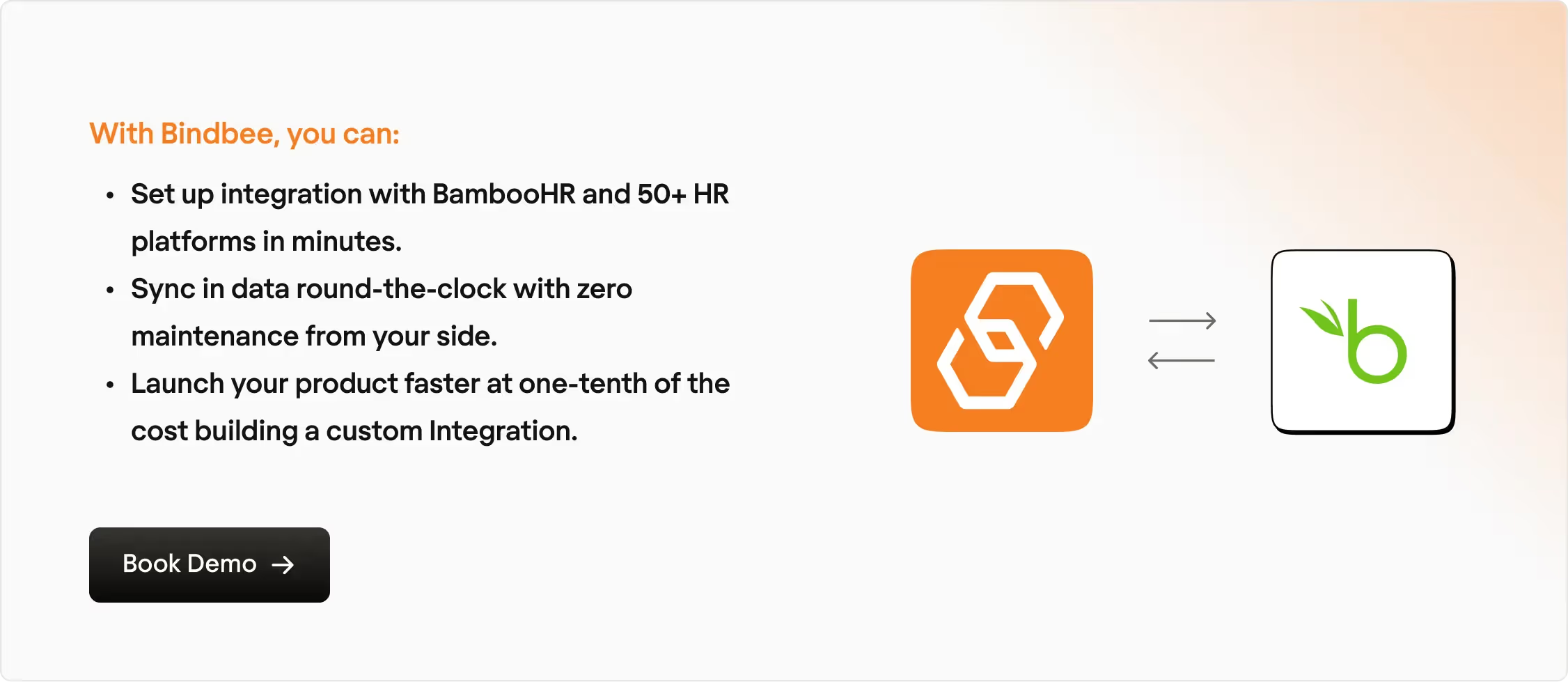
Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.